FPS (Frames Per Second) is a value that represents the number of frames that the computer renders in one second.
Steps
- Create script FPS.cs
using System.Collections;
using TMPro;
using UnityEngine;
[RequireComponent(typeof(TMP_Text))]
public class FPS : MonoBehaviour
{
private TMP_Text _fpsText;
private void Start()
{
_fpsText = GetComponent<TMP_Text>();
StartCoroutine(FramesPerSecond());
}
private IEnumerator FramesPerSecond()
{
while (true)
{
int fps = (int) (1f / Time.deltaTime);
DisplayFPS(fps);
yield return new WaitForSeconds(0.2f);
}
}
private void DisplayFPS(float fps)
{
_fpsText.text = $"{fps} FPS";
}
}
- Add Text Mesh Pro object to a Scene.
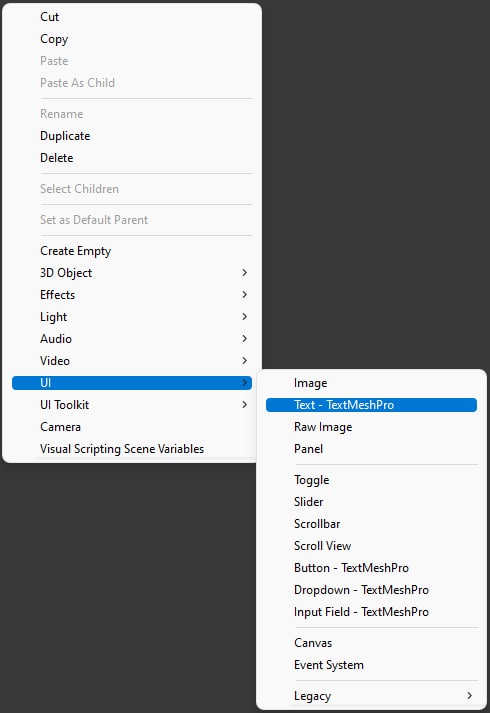
- Drag and drop FPS.cs to Text Mesh Pro in the Scene.
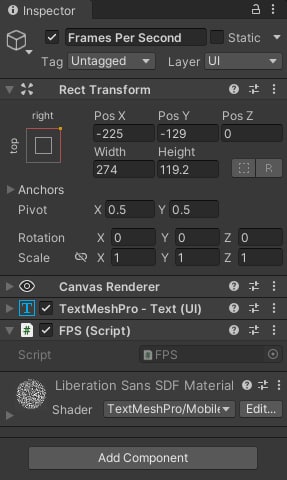
Result
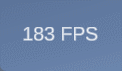
Now FPS will be displayed on a screen.