Destruction of game objects is an important element in the game. Killing the enemy, destroying the object to avoid the accumulation of debris in the scene.
Steps
- Create script DestroyObject.cs
using UnityEngine;
public class DestroyObject : MonoBehaviour
{
private void DestroyGameObject()
{
Destroy(gameObject); // Kill object on method call
}
private void DestroyComponent()
{
Destroy(GetComponent<Collider>()); // Remove collider from the game object
}
private void DestroyDelayed()
{
Destroy(gameObject, 3f); // Kill object after 3 seconds
}
private void DestroyScript()
{
Destroy(this); // Remove script from the game object
}
}
- Add script to gameobject.
- Press Play, the game object will be destroyed
Result
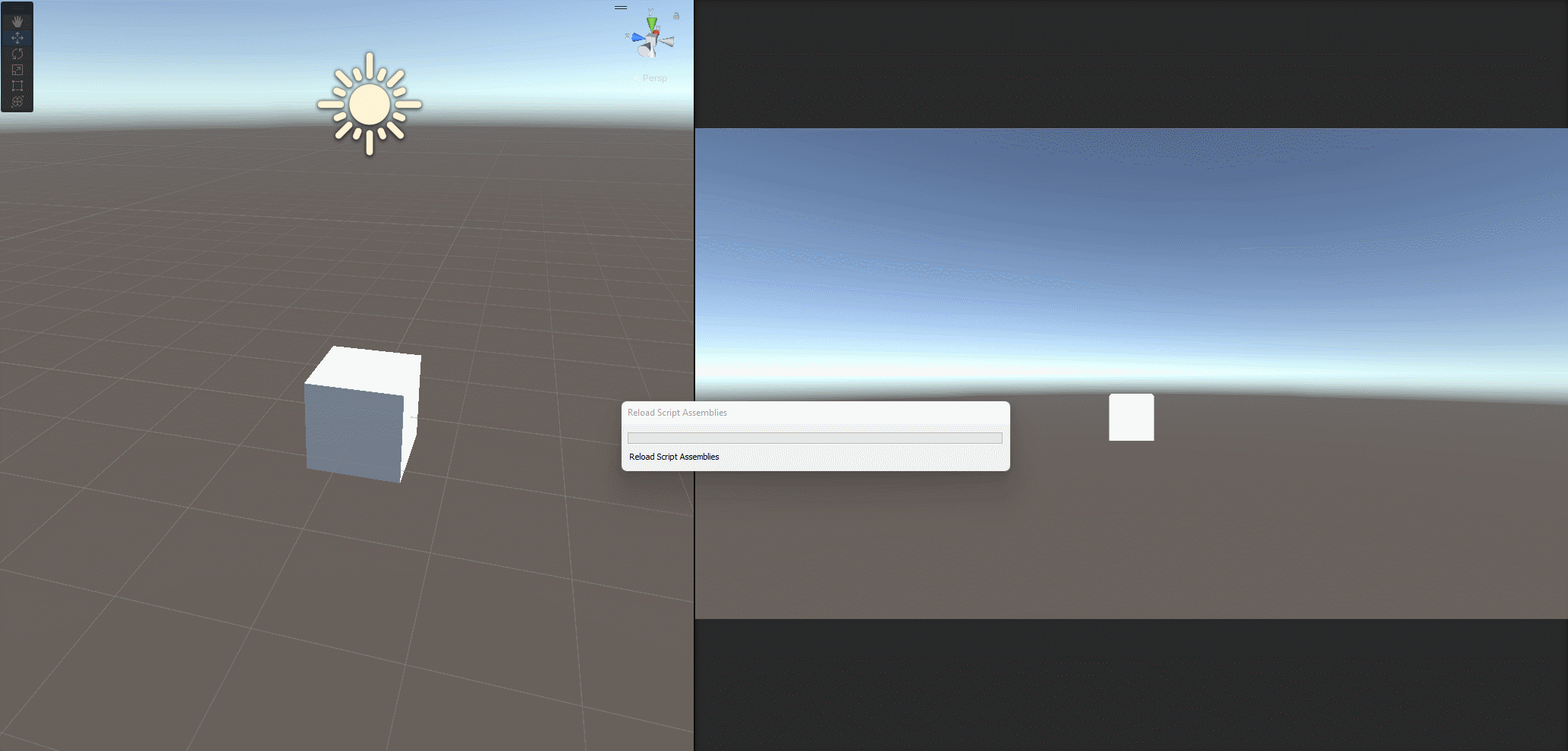