The loading bar is a convenient visual design for determining how much is left to load the game
Steps
- Create script LoadingBar.cs
using System.Collections;
using TMPro;
using UnityEngine;
using UnityEngine.SceneManagement;
using UnityEngine.UI;
public class LoadingBar : MonoBehaviour
{
[SerializeField] private Slider _slider;
[SerializeField] private TMP_Text _load;
private void Start()
{
StartCoroutine(LoadScene());
}
private IEnumerator LoadScene()
{
AsyncOperation operation = SceneManager.LoadSceneAsync(1);
operation.allowSceneActivation = false;
while (!operation.isDone)
{
float progress = Mathf.Clamp01(operation.progress / .9f);
_slider.value = progress;
_load.text = $"{progress * 100}%";
if (progress == 1)
{
_load.text = "Press [Space] to start";
if (Input.GetKeyDown(KeyCode.Space))
{
operation.allowSceneActivation = true;
}
}
yield return null;
}
}
}
- Create two scenes Loading and Main
- Add two scenes to Scene is Build File > Build Settings > Add Open Scenes
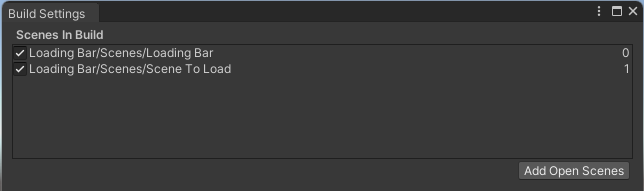
- Create a Slider customize to your taste.
- Add to Text Mesh Pro Slider
- Add script LoadingBar.cs to Slider

Result
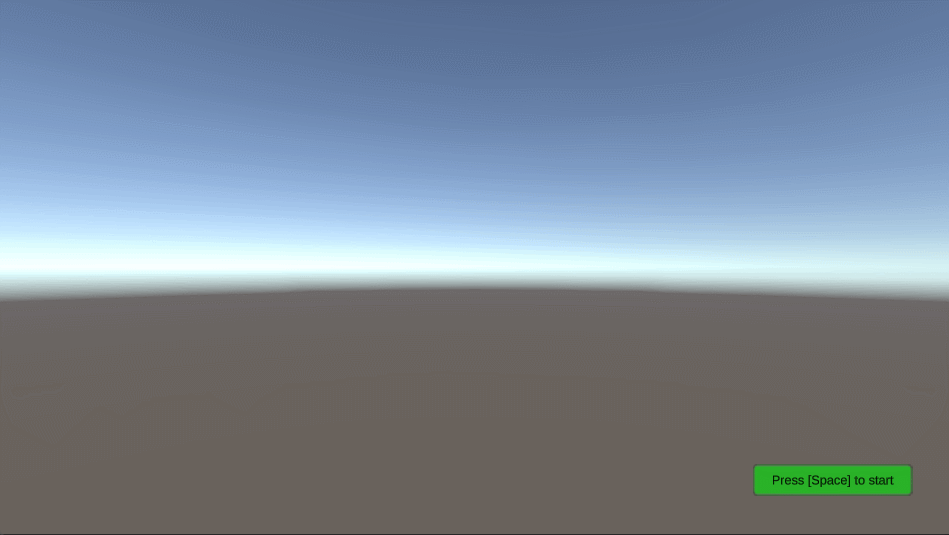