Unity provides various ways to save game data. One way involves Unity’s built-in PlayerPrefs system. Give a value to the key, call Save. Another way to save is JSON.
Steps
- Create scritps Storage.cs, Building.cs, Construction.cs
using Newtonsoft.Json;
using System.Collections;
using System.Collections.Generic;
using System.IO;
using UnityEngine;
public class Storage : MonoBehaviour
{
private const string _buildFileName = "Build.json";
private const string _buildsFileName = "Builds.json";
public static void Save(Building building)
{
string json = JsonConvert.SerializeObject(building, Formatting.Indented, new JsonSerializerSettings { ReferenceLoopHandling = ReferenceLoopHandling.Ignore });
File.WriteAllText($"{Application.persistentDataPath}/{_buildFileName}", json);
}
public static void Save(List<Building> buildings)
{
string json = JsonConvert.SerializeObject(buildings, Formatting.Indented, new JsonSerializerSettings { ReferenceLoopHandling = ReferenceLoopHandling.Ignore });
File.WriteAllText($"{Application.persistentDataPath}/{_buildsFileName}", json);
}
public static void Load(ref Building building)
{
string file = $"{Application.persistentDataPath}/{_buildFileName}";
if (ValidateFile(file))
{
building = JsonConvert.DeserializeObject<Building>(File.ReadAllText(file));
}
}
public static void Load(List<Building> buildings)
{
string file = $"{Application.persistentDataPath}/{_buildsFileName}";
if (ValidateFile(file))
{
buildings = JsonConvert.DeserializeObject<List<Building>>(File.ReadAllText(file));
}
}
private static bool ValidateFile(string file)
{
if (!File.Exists(file))
{
Debug.Log("File Not Found");
return false;
}
return true;
}
}
using Newtonsoft.Json;
using UnityEngine;
[JsonObject(MemberSerialization = MemberSerialization.OptIn)]
public class Building : MonoBehaviour
{
[SerializeField] [JsonProperty("Name")] private string _name;
[SerializeField] [JsonProperty("Cost")] private int _cost;
[JsonProperty("Position")] private Vector3 _position;
private GameObject _buildingPrefab;
public string Name => _name;
public int Cost => _cost;
public Vector3 Position => _position;
private void Awake()
{
_buildingPrefab = GetComponent<GameObject>();
_position = transform.position;
}
}
using System.Collections.Generic;
using UnityEngine;
public class Construction : MonoBehaviour
{
[SerializeField] private Building _building;
[SerializeField] private List<Building> _buildings = new();
private void Start()
{
Build();
LoadBuildings();
}
private void LoadBuildings()
{
Storage.Load(ref _building);
Storage.Load(_buildings);
}
private void Build()
{
// do something...
Storage.Save(_building);
Storage.Save(_buildings);
}
}
- Create Building object in the Scene and attach Building.cs
- Create empty object in the Scene and attach Construction.cs
- Fill in the fields in Building.cs
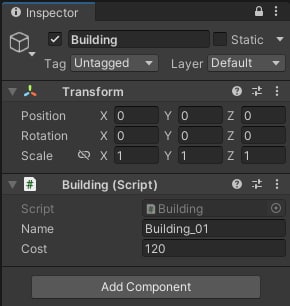
- Drag Building objects to fields Construction.cs
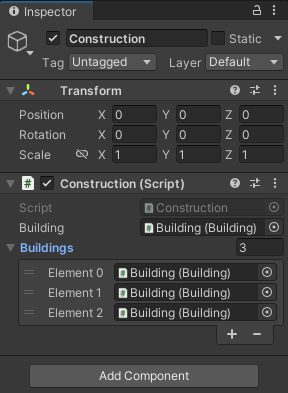
- Click Play and the data has been written to the files
Application.persistentDataPath usually points to
%userprofile%\AppData\LocalLow\<companyname>\<productname>
Then open the JSON file with text editor or your IDE to code.
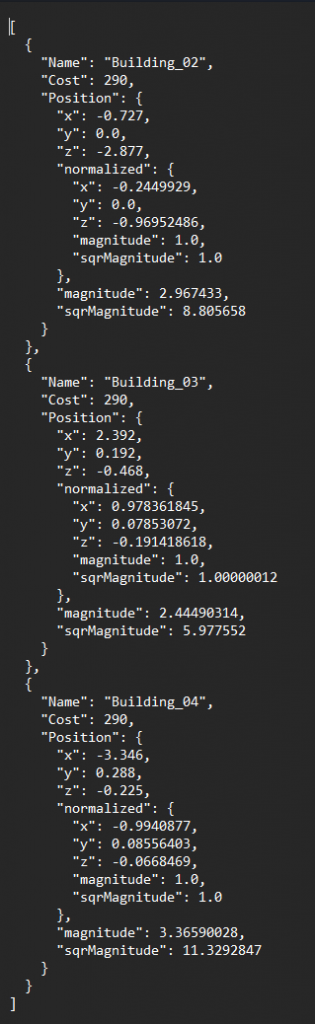