Attributes are markers that can be placed above a class, property or function in a script to indicate special behaviour.
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
[RequireComponent(typeof(Rigidbody)), SelectionBase] // The RequireComponent attribute automatically adds required components as dependencies.
// SelectionBase Add this attribute to a script class to mark its GameObject as a selection base object for Scene View picking.
public class Attributes : MonoBehaviour
{
[HideInInspector] public string value01; // Makes a variable not show up in the inspector but be serialized.
[Min(0)] public int value02; // Attribute used to make a float or int variable in a script be restricted to a specific minimum value.
[Header("Title")] public string value03; // Use this PropertyAttribute to add a header above some fields in the Inspector.
[Space(20)] public string value04; // Use this PropertyAttribute to add some spacing in the Inspector.
[Multiline] public string value05; // Attribute to make a string be edited with a multi-line textfield.
[Range(0f, 1f)] public float value06; // Attribute used to make a float or int variable in a script be restricted to a specific range.
[TextArea(5, 7)] public string value07; // Attribute to make a string be edited with a height-flexible and scrollable text area.
// Where 5 is the minimum amount of lines the text area will use.
// 7 is the maximum amount of lines the text area can show before it starts using a scrollbar.
[Tooltip("This is value")] public string value08; // Specify a tooltip for a field in the Inspector window.
[SerializeField] private string _value09; // Force Unity to serialize a private field.
[ContextMenuItem("Reset Value", "ResetValue10")] public int value10; // Use this attribute to add a context menu to a field that calls a named method.
[ContextMenu("Add To Value 02 (+1)")] // The ContextMenu attribute allows you to add commands to the context menu.
private void AddToValue02()
{
value02++;
}
private void ResetValue10()
{
value10 = 0;
}
}
RequireComponent
The RequireComponent attribute automatically adds required components as dependencies.
When you add a script which uses RequireComponent to a GameObject, the required component is automatically added to the GameObject. This is useful to avoid setup errors. For example a script might require that a Rigidbody is always added to the same GameObject. When you use RequireComponent, this is done automatically, so you are unlikely to get the setup wrong.
Note: RequireComponent only checks for missing dependencies when GameObject.AddComponent is called. This happens both in the Editor, or at runtime. Unity does not automatically add any missing dependences to the components with GameObjects that lack the new dependencies.
SelectionBase
Add this attribute to a script class to mark its GameObject as a selection base object for Scene View picking.
In the Unity Scene View, when clicking to select objects, Unity will try to figure out the best object to select for you. If you click on an object that is part of a Prefab, the root of the Prefab is selected, because a Prefab root is treated as a selection base. You can make other objects be treated as selection base too. You need to create a script class with the SelectionBase attribute, and then you need to add that script to the GameObject.
HideInInspector
Makes a variable not show up in the inspector but be serialized.
Min
Attribute used to make a float or int variable in a script be restricted to a specific minimum value.
Header
Use this PropertyAttribute to add a header above some fields in the Inspector.
Space
Use this PropertyAttribute to add some spacing in the Inspector. The spacing in pixels.
Multiline
Attribute to make a string be edited with a multi-line textfield.
Range
Attribute used to make a float or int variable in a script be restricted to a specific range.
When this attribute is used, the float or int will be shown as a slider in the Inspector instead of the default number field.
TextArea
Attribute to make a string be edited with a height-flexible and scrollable text area.
You can specify the minimum and maximum lines for the TextArea, and the field will expand according to the size of the text. A scrollbar will appear if the text is bigger than the area available.
Note: The maximum lines refers to the maximum size of the TextArea. There is no maximum to the number of lines entered by the user.
Tooltip
Specify a tooltip for a field in the Inspector window.

SerializeField
Force Unity to serialize a private field.
When Unity serializes your scripts, it only serializes public fields. If you also want Unity to serialize your private fields you can add the SerializeField attribute to those fields.
Unity serializes all your script components, reloads the new assemblies, and recreates your script components from the serialized versions. This serialization is done with an internal Unity serialization system; not with .NET’s serialization functionality.
The serialization system can do the following:
- CAN serialize public non-static fields (of serializable types)
- CAN serialize nonpublic non-static fields marked with the SerializeField attribute.
- CANNOT serialize static fields.
- CANNOT serialize properties.
Serializable types
Unity can serialize fields of the following types:
- All classes inheriting from UnityEngine.Object, for example GameObject, Component, MonoBehaviour, Texture2D, AnimationClip.
- All basic data types, such as int, string, float, bool.
- Some built-in types, such as Vector2, Vector3, Vector4, Quaternion, Matrix4x4, Color, Rect, LayerMask.
- Arrays of a serializable type
- Lists of a serializable type
- Enums
- Structs
Note: If you put one element in a list (or array) twice, when the list gets serialized, you’ll get two copies of that element, instead of one copy being in the new list twice.
Note: If you want to serialize a custom Struct field, you must give the Struct the [System.Serializable] attribute.
Hint: Unity won’t serialize Dictionary, however you could store a List<> for keys and a List<> for values, and sew them up in a non serialized dictionary on Awake(). This doesn’t solve the problem of when you want to modify the dictionary and have it “saved” back, but it is a handy trick in a lot of other cases.
ContexMenu
The ContextMenu attribute allows you to add commands to the context menu.
In the inspector of the attached script. When the user selects the context menu, the function will be executed.
This is most useful for automatically setting up Scene data from the script. The function has to be non-static.

ContextMenuItem
Use this attribute to add a context menu to a field that calls a named method.
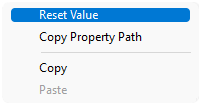
Result
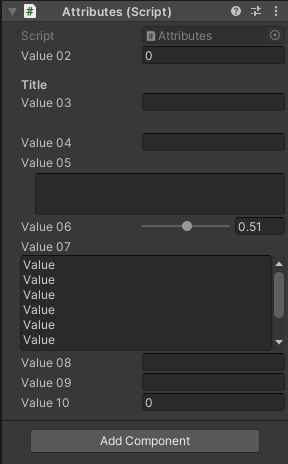