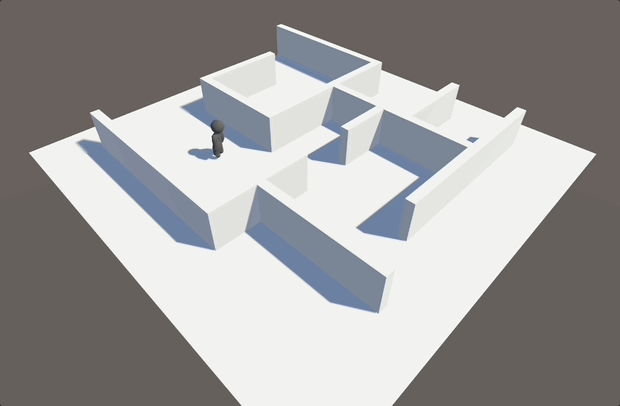
NavMeshAgent is a component in Unity 3D that provides automatic pathfinding for game characters based on a navigation mesh. This component allows you to create “smart” characters that can automatically move towards a specified point, avoiding obstacles in their path.
Steps
- Create script PlayerController.cs
using System;
using UnityEngine;
using UnityEngine.AI;
[RequireComponent(typeof(NavMeshAgent))]
public class PlayerController : MonoBehaviour
{
private enum AnimationKeys
{
Walking
}
private NavMeshAgent _agent;
private Animator _animator;
private void Start()
{
_agent = GetComponent<NavMeshAgent>();
_animator = GetComponent<Animator>();
}
private void Update()
{
if (Input.GetKeyDown(KeyCode.Mouse0))
{
RaycastHit hit;
if (Physics.Raycast(Camera.main.ScreenPointToRay(Input.mousePosition), out hit, Mathf.Infinity))
{
_agent.SetDestination(hit.point);
_animator.SetBool(AnimationKeys.Walking.ToString(), true);
}
}
if (!_agent.pathPending && _agent.remainingDistance < 0.1f)
{
_animator.SetBool(AnimationKeys.Walking.ToString(), false);
}
}
}
- To open the Navigator menu, go to Windows > AI > Navigation.
- Go to the Object tab and select the ground. Check the box next to Navigation Static and choose the Walkable option in Navigation Area.
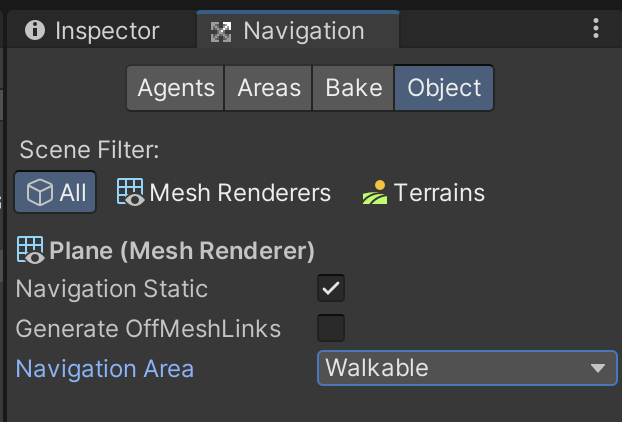
- Go to the Object tab and select the walls. Check the box next to Navigation Static and choose the No Walkable option in Navigation Area.
- Go to the Bake tab and set up parameters for the player.
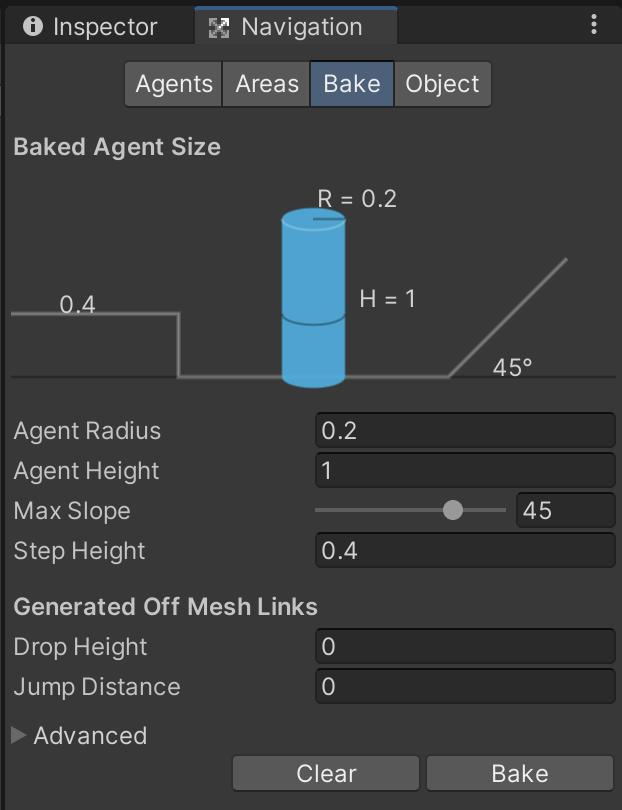
- Click on the Bake button.
After completing all the steps, you should see blue boundaries where the player can walk.
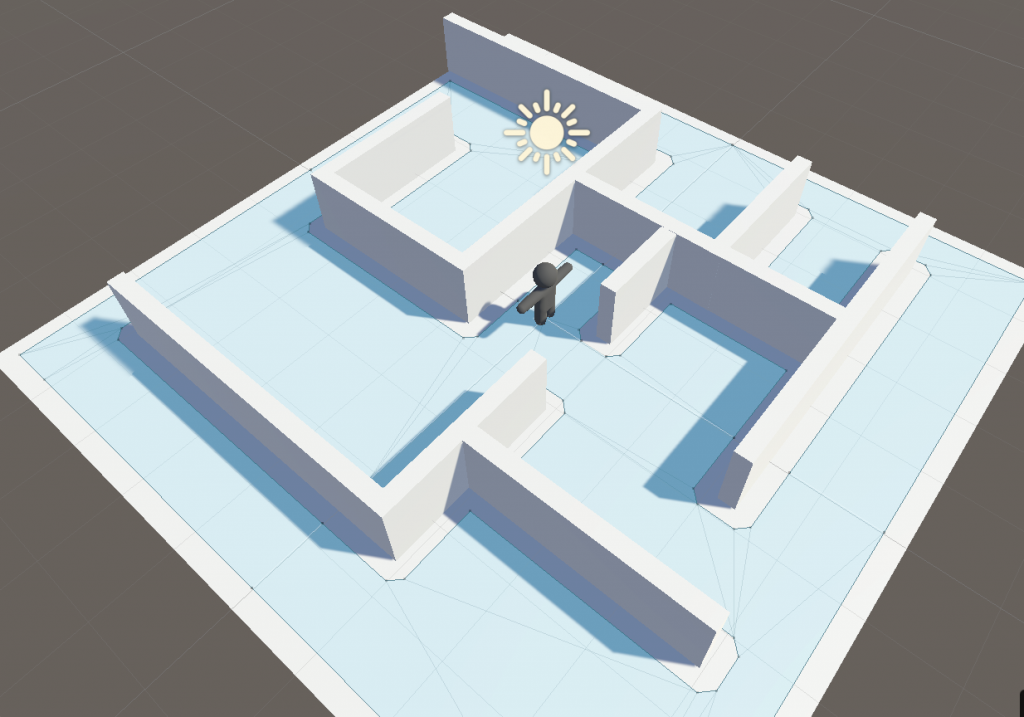
Next steps
- Add script PlayerController.cs and NavMeshAgent component to player
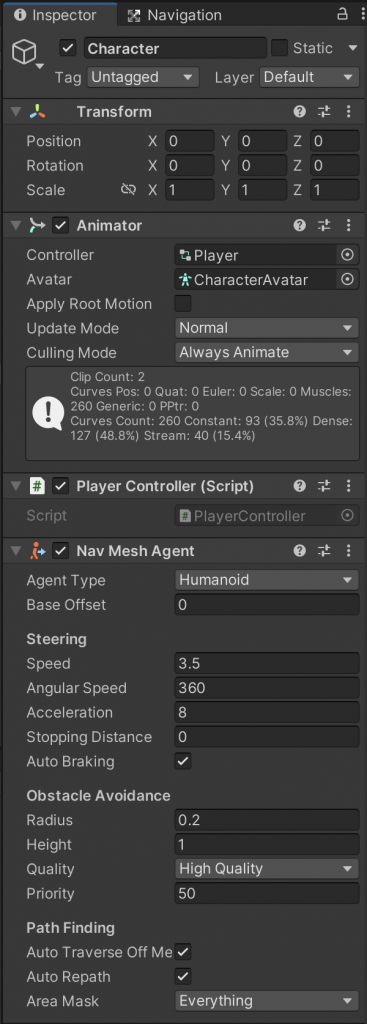